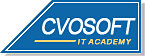
✒️SAP Fiori La utilización de los controles en la UI
SAP Fiori La utilización de los controles en la UI
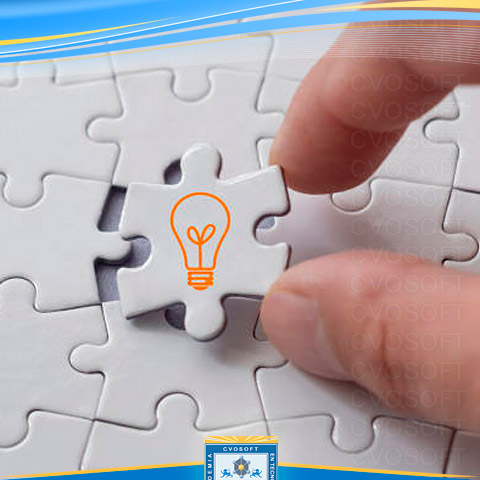
1 | SAPUI5 UI Controls
What are SAPUI5 Controls?
SAPUI5 controls are user interface elements used for interactive operations, either passively or actively. Each control defines how a specific area of the screen appears and behaves.
Overview of SAPUI5 Controls
In SAPUI5, controls are categorized into mobile-focused UI controls and desktop-centric UI controls. It's recommended to use mobile-focused controls as desktop controls in the sap.ui namespace are deprecated.
Key Components of Controls:
- Control Name: Each control has a name consisting of a library name and control name, like sap.m.Table for creating a table.
- Control Metadata: Defines properties, events, aggregations, and associations of a control. Properties modify appearance or data relations. Controls are defined by their metadata, which forms the control's public API.
- Aggregations: Controls can contain other controls or elements. Aggregations serve as containers or layout controls where secondary controls can be added.
- Associations: These connect controls using IDs rather than references. Associated controls are not part of or child elements of an aggregation.
- Events: Higher-level semantic events tied to control functionality, distinct from browser-level events like click. For example, press event for a button.
- Elements: Configurations within controls, not independently usable, without their renderer.
2 | Creating a SAPUI5 Table Control
In SAPUI5, tables are essential for displaying structured data effectively. They provide a clear and organized way to present information, making them a cornerstone in user interfaces. The recommended table control for responsive applications is sap.m.Table, as opposed to sap.ui.table.Table, which is not responsive.
Elements of SAPUI5 Table Controls
- Columns Aggregation: Tables in SAPUI5 consist of columns defined within the columns aggregation. Each column determines the structure and appearance of the data displayed in the table. Columns can include various properties and configurations, such as width and visibility settings.
<Table id="myTable" items="{/Products}"><columns><Column><Text text="Product Name"/></Column><Column><Text text="Price"/></Column><!-- Additional columns as needed --></columns></Table>
- Data Binding: Data binding is fundamental for dynamically populating tables with data fetched from models. It allows seamless synchronization between the data source and the UI controls, ensuring that any changes in the data reflect immediately in the table.
// Setting model and bindingvar oModel = new sap.ui.model.json.JSONModel();oModel.loadData("path/to/products.json");this.getView().setModel(oModel);
// Binding items to the tablevar oTable = this.getView().byId("myTable");oTable.bindItems({path: "/Products",template: new sap.m.ColumnListItem({cells: [new sap.m.Text({ text: "{ProductName}" }),new sap.m.Text({ text: "{Price}" })// Additional cells for other properties]})});
- Responsive Design: SAPUI5 provides responsive features out-of-the-box, ensuring that tables adapt fluidly to different screen sizes and orientations. This is achieved by setting properties like responsive and fullScreenOptimized to true in controls such as ObjectHeader.
Example: Creating a JSON Model
In SAPUI5 applications, models serve as data sources for binding to UI controls. JSON models are commonly used for smaller data sets due to their simplicity and ease of integration.
- Model Loading: JSON models are typically loaded during the initialization phase (init() method) of the controller. This ensures that data is available when the view is rendered.
- Manifest.json Configuration: To streamline model loading, configuration in manifest.json defines the model's data source (dataSource) and type. This setup automates model loading, minimizing explicit implementation.
{"sap.app": {"dataSources": {"products": {"uri": "path/to/products.json","type": "JSON"}}},"sap.ui5": {"models": {"products_json": {"dataSource": "products","type": "sap.ui.model.json.JSONModel"}}}}
3 | Using Models in SAPUI5
Models in SAPUI5 facilitate seamless data binding between backend data sources and UI controls. They ensure that changes in data are reflected instantly in the application's interface, providing a robust foundation for interactive applications.
Data Binding Essentials
- Model Attachment: Models can be attached at different levels within the application hierarchy:
- Application Level: sap.ui.getCore().setModel(model);
- View Level: this.getView().setModel(model);
- Control Level: sap.ui.getCore().byId("__xmlview0--Table1").setModel(model);
The closest model to a control takes precedence, ensuring targeted data binding.
- Event Handling: SAPUI5 models support events such as attachRequestCompleted, enabling applications to react to model loading events asynchronously. This ensures UI responsiveness and data integrity.
- Usage of Models: Models are retrieved in views using this.getOwnerComponent().getModel("products_json"), leveraging predefined models for seamless data integration.
Example: Binding Models in Views
In the view, binding models involves specifying the model name and property paths to synchronize UI controls with data.
<Table id="__xmlview0--Table1" items="{products_json>/Products}"><columns><Column><Text text="Product Name"/></Column><Column><Text text="Price"/></Column><!-- Additional columns as needed --></columns></Table>
4 | Creating Controls
Creating the Input Control
Examples of the Input Control: We can find an example of this control at the following URL:
sapui5.hana.ondemand.com/sdk/#/entity/sap.m.Input
The API details can be viewed at: sapui5.hana.ondemand.com/sdk/#/api/sap.m.Input
To use the Input control in our view, we must include the "sap.m" reference.
The properties of this control are documented in the SAP API. Let's focus on those used in this example:
- id attribute: Specifies a unique identifier for an HTML element, used for CSS styling and JavaScript DOM manipulation.
- value attribute: Specifies the value of an element.
- maxLength attribute: Sets the maximum length allowed for input.
- class attribute: Assigns a CSS class for styling purposes.
- placeholder attribute: Provides a hint to the user about what to enter.
- showSuggestion attribute: Enables dynamic suggestions based on user input.
- suggestionItems attribute: Specifies which field of the model provides suggestions.
Creating the Select Control
The SAPUI5 Select control displays a menu of options.
You can explore the control in the SAP API reference:
sapui5.hana.ondemand.com/sdk/#/entity/sap.m.Select
Let's analyze the properties used in our example:
- selectedKey attribute: Stores the value of the selected item.
- items attribute: Defines the values displayed in the dropdown menu.
- forceSelection attribute: Specifies if selection is restricted to list items.
- visible attribute: Controls the visibility of the control.
Creating the Label Control
The Label control serves as a tag for a UI element. It can be associated with another control using the 'for' attribute or by nesting it.
Explore the Label control in the SAP API:
sapui5.hana.ondemand.com/sdk/#/entity/sap.m.Label
To associate a label, use the 'labelFor' property.
Creating the Button Control
The Button control is used to create buttons in the user interface.
Explore the Button control in the SAP API:
sapui5.hana.ondemand.com/sdk/#/entity/sap.m.Button
Buttons can be associated with events in the view's controller. For example, handling user clicks to filter or reset data.
5 | Events
Events are the execution of functions in response to an action and are sent to notify the code of interesting things that have happened. The function is commonly referred to as a "listener" or a "handler," with both terms used interchangeably.
Custom Event Handling
This pattern can be easily followed using completely customized code. In this case, we will add an event when the user clicks the button to search for records that meet the filter criteria, and another event when we clear the filter.
Implementing Event Logic
Using the Button control, let's explore the event logic for filtering when the user enters a product name or a currency. First, we retrieve the model data using this.getView().getModel().getData(). Then, we check if the CurrencyCode field is filled. If so, we create a filter using the "EQ" operator for equality and compare it with the entered value in the UI. For the description field, we use the "Contains" operator to match the string entered, removing white spaces with the JS trim() method.
Using Filters
To apply filters, we need to include two libraries in our project within sap.ui.define:
sap.ui.define(["sap/ui/model/Filter","sap/ui/model/FilterOperator"],
Case Sensitivity in OData Filters
Filters in OData services are case-sensitive. If a case-insensitive search is preferred, it can be implemented in the controller logic.
Debugging Model Data
In the debug console, we can observe how getModel(ModelName).getData() returns the model data.
Binding and Filtering
To obtain and filter list data, we use this.getView().byId("idProductoTable") to get the table control by its XML view ID. The binding information for the table rows is obtained using getBinding("items").
Applying Filters
Filters are applied to the table using oBinding.filter(filters). For example, filtering by a product and its currency yields specific results.
Clearing Filters
To clear filters, we also have a press event on the button to perform that action. The button logic involves using setProperty to set model-bound fields to blank, effectively clearing the view.
6 | Context and Messages
Context Context is a pointer to an object in the model data. Each function invocation has a scope and an associated context. Scope primarily deals with function-based access to variables, unique to each invocation. Context always refers to the value of the this keyword, which points to the object that "owns" the currently executing code.
The method getBindingContext() provides us with the binding context applied to an element's control. When we bind elements (context binding) to a control, using getBindingContext() returns the specific context details applied to that control.
In our ongoing example using the table control, the data table we're passing results in multiple rows. Internally, SAPUI5 APIs perform context binding for each generated row. Therefore, when we use the event handler method on item click, we can call item.getBindingContext(), which gives us the binding context for that object within the given model name. Additionally, item.getBindingContext().getObject() allows access to the data.
For an example, let's code the click event of a table item. Upon clicking, we'll display a message with object data, as shown in the following:
To display a message with the supplier's name, we use the sap.m.MessageToast library with the show method. MessageToast is a small, non-disruptive pop-up window for success messages that automatically disappears after a few seconds.
 
 
 
Sobre el autor
Publicación académica de Jaime Eduardo Gomez Arango, en su ámbito de estudios para la Carrera Consultor en SAP Fiori.
Jaime Eduardo Gomez Arango
Profesión: Ingeniero de Sistemas y Computación - España - Legajo: SW34C
✒️Autor de: 149 Publicaciones Académicas
🎓Cursando Actualmente: Consultor en SAP Fiori
🎓Egresado de los módulos:
Disponibilidad Laboral: FullTime
Presentación:
Ingeniero de sistemas y computación con 8 años de experiencia el desarrollo frontend & backend (react/node) y en cloud (aws), actualmente desarrollando habilidades en sap btp, ui5, abap y fiori.
Certificación Académica de Jaime Gomez