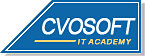
✒️Las funciones más utilizadas en SAPUI5
Las funciones más utilizadas en SAPUI5

1 | Key Functions in SAPUI5
We will explore some of the most commonly used functions and methods in SAPUI5, crucial for developing SAP Fiori applications.
Accessing Core Information
One important aspect of developing SAPUI5 applications is accessing different elements from any point in the application. For instance, you might need to retrieve a control's value from a view or access data from a model to populate a combobox.
- sap.ui.getCore(): This function returns an instance of the core of the application. The core can store global information, models, and more. It is the most powerful function at the application level.
var language = sap.ui.getCore().getConfiguration().getLanguage();console.log("Logged in language: " + language);
- sap.ui.getCore().byId(id): This function retrieves a SAPUI5 control instance created with a specific ID.
var myControl = sap.ui.getCore().byId("myControlId");
- this.getView().byId(id): Used in a view controller to get a control by its ID.
var myControl = this.getView().byId("myControlId");
- sap.ui.getCore().applyChanges(): This function applies and renders changes immediately to the UI5 controls. If not called, the DOM will not update.
sap.ui.getCore().applyChanges();
- jQuery.sap.domById(id): Retrieves an HTML element with a specific ID.
var htmlElement = jQuery.sap.domById("myHtmlId");
- jQuery.sap.byId(id): Returns the jQuery object of the DOM element with the specified ID, useful for visual effects.
var jqElement = jQuery.sap.byId("myHtmlId");jqElement.css("color", "red");
2 | Methods for Arrays in JavaScript
JavaScript arrays are special objects that store collections of data. Arrays in SAP Fiori applications are frequently used to manage and manipulate data.
- The find Method: This method executes a callback function for each array element and returns the first element that satisfies the callback function's condition.
var products = [{ id: 1, price: 20 }, { id: 2, price: 22 }];var product = products.find(product => product.price === 22);console.log(product); // { id: 2, price: 22 }
- The forEach Method: This method executes a callback function for each array element.
products.forEach(product => console.log(product.id));
- The map Method Similar to forEach, but returns a new array with the results of the callback function.
var prices = products.map(product => product.price);console.log(prices); // [20, 22]
- The reduce Method: This method executes a reducer function on each array element, resulting in a single output value.
var total = products.reduce((sum, product) => sum + product.price, 0);console.log(total); // 42
- The filter Method: This method creates a new array with all elements that pass the test implemented by the provided function.
var expensiveProducts = products.filter(product => product.price > 20);console.log(expensiveProducts); // [{ id: 2, price: 22 }]
- The sort Method: This method sorts the elements of an array in place and returns the sorted array.
products.sort((a, b) => a.price - b.price);console.log(products); // [{ id: 1, price: 20 }, { id: 2, price: 22 }]
3 | Inserting or Removing Array Elements
Adding and Removing Elements at the Start
- shift(): Removes the first element of an array.
var firstElement = products.shift();console.log(firstElement); // { id: 1, price: 20 }
- unshift('New Element'): Adds a new element at the beginning of an array.
products.unshift({ id: 0, price: 18 });console.log(products); // [{ id: 0, price: 18 }, { id: 2, price: 22 }]
Adding and Removing Elements at the End
- push('New Element'): Adds a new element to the end of an array.
products.push({ id: 3, price: 25 });console.log(products); // [{ id: 0, price: 18 }, { id: 2, price: 22 }, { id: 3, price: 25 }]
- pop(): Removes the last element of an array.
var lastElement = products.pop();console.log(lastElement); // { id: 3, price: 25 }
Array Index
Array indices start at 0. Accessing an undefined index returns undefined.
console.log(products[0]); // { id: 0, price: 18 }
Finding Index and Checking Inclusion
- indexOf: Returns the first index at which a given element can be found.
var index = products.indexOf(products[1]);console.log(index); // 1
- includes: Checks if an array includes a certain value.
var includesProduct = products.includes(products[1]);console.log(includesProduct); // true
4 | Loops
Loops, also known as iterations, allow repetitive execution of code, often used for traversing arrays.
- The for Loop: The most common loop for traversing arrays.
for (var i = 0; i < products.length; i++) {console.log(products[i].id);}
- The while Loop: Executes code as long as a specified condition is true.
var i = 0;while (i < products.length) {console.log(products[i].id);i++;}
- The do...while Loop: Executes code at least once before checking the condition.
var i = 0;do {console.log(products[i].id);i++;} while (i < products.length);
- The length Property: Retrieves the number of elements in an array.
console.log(products.length); // 2
5 | Literal Objects
Literal objects store more complex data than arrays, consisting of properties and methods.
Defining Literal Objects
var product = {id: 1,price: 20,name: "Product 1"};
JSON Parsing and Stringifying
- JSON.parse(): Converts a JSON string into a JavaScript object.
var jsonString = '{"id":1,"price":20}';var product = JSON.parse(jsonString);console.log(product); // { id: 1, price: 20 }
- JSON.stringify(): Converts a JavaScript object into a JSON string.
var jsonString = JSON.stringify(product);
console.log(jsonString); // '{"id":1,"price":20}'
6 | Methods
Methods are functions associated with objects, used to perform actions like modifying properties or handling events.
Regular Methods and Event Handlers
Regular methods can be public or private, while event handlers start with "on" and handle specific events.
var product = {id: 1,price: 20,displayInfo: function() {console.log(this.id + " - " + this.price);}};product.displayInfo(); // 1 - 20
The placeAt Method in JavaScript
Used to add content to a specified HTML element.
var oText = new sap.m.Text({ text: "Hello World" });oText.placeAt("content");
 
 
 
Sobre el autor
Publicación académica de Jaime Eduardo Gomez Arango, en su ámbito de estudios para la Carrera Consultor en SAP Fiori.
Jaime Eduardo Gomez Arango
Profesión: Ingeniero de Sistemas y Computación - España - Legajo: SW34C
✒️Autor de: 149 Publicaciones Académicas
🎓Cursando Actualmente: Consultor en SAP Fiori
🎓Egresado de los módulos:
Disponibilidad Laboral: FullTime
Presentación:
Ingeniero de sistemas y computación con 8 años de experiencia el desarrollo frontend & backend (react/node) y en cloud (aws), actualmente desarrollando habilidades en sap btp, ui5, abap y fiori.
Certificación Académica de Jaime Gomez