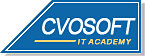
✒️Los conceptos de enlace de datos en SAPUI5
Los conceptos de enlace de datos en SAPUI5
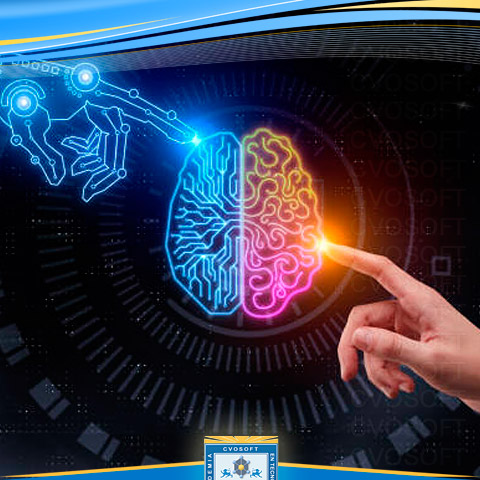
1 | The Concept of Data Binding
Benefits of Binding
Data binding is a fundamental technique in SAPUI5 that allows linking model data to UI controls. Efficient use of this technique can significantly reduce the amount of code needed in a SAPUI5 application, simplifying development and maintenance.
Types of Binding
There are several types of binding modes in SAPUI5 to handle different scenarios:
- One-way binding
- Changes in the model are reflected in the UI, but changes made in the UI do not affect the model.
- Example: Updating the price of a product in the model automatically displays the new price in the UI.
- Two-way binding
- Ensures that both the model and the UI are always synchronized. Any change in one is automatically reflected in the other.
- Example: If the user updates their address in the UI, the model is also updated with the new address.
- One-time binding
- Model values are transferred to the UI only once and do not update afterward.
- Example: Displaying a welcome message that does not change after initially being shown.
- Aggregation Binding
- Aggregation binding is relevant for controls that have multiple child elements, such as tables, lists, or dropdowns. This type of binding allows child controls to be automatically created based on the model data.
- Example: Binding a table to a JSON model containing a list of products. Each row of the table will be automatically generated for each product in the model.
2 | Creating a Project and Binding
Creating a Project
We will create a SAPUI5 project where we configure a user interface (UI) with two input fields for the first name and last name, and a text field to display the full name (a concatenation of the first and last names). When the first name or last name changes, the full name field will automatically update.
Practical Example
- Create an MTA Project
- Select a SAPUI5 application.
- We do not connect to a database in this example.
- Create the XML View
<mvc:ViewcontrollerName="myApp.controller.Main"xmlns:mvc="sap.ui.core.mvc"xmlns="sap.m"><VBox><Input id="firstName" placeholder="First Name"/><Input id="lastName" placeholder="Last Name"/><Text id="fullName" text="{= ${firstName} + ' ' + ${lastName} }"/></VBox></mvc:View>
- Controller
sap.ui.define(["sap/ui/core/mvc/Controller"], function (Controller) {"use strict";return Controller.extend("myApp.controller.Main", {onInit: function () {var oModel = new sap.ui.model.json.JSONModel({firstName: "",lastName: ""});this.getView().setModel(oModel);}});});
3 | The Element Binding Technique
Element Binding
The element binding technique allows a SAPUI5 control to be linked to a specific data element in a model, creating a binding context for the control and all its children.
Practical Example
- JSON Model
var oModel = new sap.ui.model.json.JSONModel({suppliers: [{name: "Supplier 1",products: [{ name: "Product A" },{ name: "Product B" }]},{name: "Supplier 2",products: [{ name: "Product C" },{ name: "Product D" }]}]});
- XML View
<mvc:ViewcontrollerName="myApp.controller.Main"xmlns:mvc="sap.ui.core.mvc"xmlns="sap.m"><List items="{/suppliers}"><items><ObjectListItem title="{name}" press="onSupplierPress"/></items></List><Table id="productsTable" items="{}"><columns><Column><Text text="Product Name"/></Column></columns><items><ColumnListItem><cells><Text text="{name}"/></cells></ColumnListItem></items></Table></mvc:View>
- Controller
sap.ui.define(["sap/ui/core/mvc/Controller"], function (Controller) {"use strict";return Controller.extend("myApp.controller.Main", {onInit: function () {var oModel = new sap.ui.model.json.JSONModel({suppliers: [{name: "Supplier 1",products: [{ name: "Product A" },{ name: "Product B" }]},{name: "Supplier 2",products: [{ name: "Product C" },{ name: "Product D" }]}]});this.getView().setModel(oModel);},onSupplierPress: function (oEvent) {var oContext = oEvent.getSource().getBindingContext();this.getView().byId("productsTable").setBindingContext(oContext);}});});
4 | How to Perform Advanced Operations like Filtering, Sorting, and Grouping?
Filtering
Filtering allows limiting the data displayed in a list or table based on certain criteria.
Practical Example
- Add Filter in the Controller
var oFilter = new sap.ui.model.Filter("name", sap.ui.model.FilterOperator.Contains, "Product A");var oList = this.getView().byId("productsTable");var oBinding = oList.getBinding("items");oBinding.filter([oFilter]);
Sorting and Grouping
Sorting arranges the data in ascending or descending order, while grouping organizes it based on a specific property.
Practical Example
- Directly in the view
<mvc:ViewcontrollerName="myApp.controller.Main"xmlns:mvc="sap.ui.core.mvc"xmlns="sap.m"><VBox><List id="productList" items="{path: '/products',sorter: [{path: 'category',group: true},{path: 'price',descending: true}]}"><items><StandardListItemtitle="{name}"description="{price}"info="{category}" /></items></List></VBox></mvc:View>
- Sorting in the Controller
var oSorter = new sap.ui.model.Sorter("name", false); // false for ascendingvar oList = this.getView().byId("productsTable");var oBinding = oList.getBinding("items");oBinding.sort([oSorter]);
- Grouping in the Controller
var oSorter = new sap.ui.model.Sorter("supplier", false, true); // true for groupingvar oList = this.getView().byId("productsTable");var oBinding = oList.getBinding("items");oBinding.sort([oSorter]);
5 | Model Inheritance
In SAPUI5, a model assigned to a higher level in the view hierarchy is automatically inherited by all lower levels unless overridden at a lower level.
Practical Example
- Define Models in manifest.json
"models": {"": {"dataSource": "mainService"},"i18n": {"type": "sap.ui.model.resource.ResourceModel","settings": {"bundleName": "myApp.i18n.i18n"}}}
- Assign a Named Model in the Controller
var oView = this.getView();var oModel = new sap.ui.model.json.JSONModel(data);oView.setModel(oModel, "mainModel");
Resource Model
A resource model is used for internationalization, linking i18n files to obtain translatable texts.
Practical Example
- Create an Instance of the Resource Model
var oResourceModel = new sap.ui.model.resource.ResourceModel({bundleName: "myApp.i18n.i18n"});
this.getView().setModel(oResourceModel, "i18n");
 
 
 
Sobre el autor
Publicación académica de Jaime Eduardo Gomez Arango, en su ámbito de estudios para la Carrera Consultor en SAP Fiori.
Jaime Eduardo Gomez Arango
Profesión: Ingeniero de Sistemas y Computación - España - Legajo: SW34C
✒️Autor de: 149 Publicaciones Académicas
🎓Cursando Actualmente: Consultor en SAP Fiori
🎓Egresado de los módulos:
Disponibilidad Laboral: FullTime
Presentación:
Ingeniero de sistemas y computación con 8 años de experiencia el desarrollo frontend & backend (react/node) y en cloud (aws), actualmente desarrollando habilidades en sap btp, ui5, abap y fiori.
Certificación Académica de Jaime Gomez